JavaScript ES6 concepts and principles are fundamental. Nowadays new modern javascript libraries and frameworks use these concepts. ES6 concepts, covering variables and data types, functions, arrays and objects, control flow and loops, error handling, scope and closures, and DOM manipulation. Each section provides an in-depth explanation with examples to help you understand these fundamental aspects of JavaScript. Nowadays React Angular and other javascript technologies are using these core practices. By mastering the fundamentals of JavaScript ES6, you’ll be equipped with the knowledge and skills to write clean, maintainable code and tackle more advanced JavaScript concepts with confidence. So, let’s dive in and unlock the power of JavaScript ES6 together!
Difference between var, let & const
By using var
old way of defining variables. But javascript still maintains this type of syntax so, that old written code can also be executed and run by language because after an update each code updates a crucial thing.
var p_name = "jhon";
console.log(p_name); // will print jhon
p_name = "Rahul";
console.log(p_name); // will print Rahul
var p_name="Ramesh";
console.log(p_name);
In the above code, you can see it different way of defining var. The var is globally scoped so, you can define its top, and can be modified anywhere wherever required let me explain by example.
var username = "Jhon";
var age = 24;
if (age > 23) {
var usename = "Mosh";
}
console.log(usename) // Will print "Mosh"
In the above example, you can see that we have defined a username and in the if statement block is modified and can be accessed outside the block and able to print it. But in the case of let and const this will not happen because both are block scoped so, will able to access within the block the same thing let me explain to you by example.
let username = "Jhon";
let age = 24;
if (age > 23) {
let usename = "Mosh";
}
console.log(usename) // Will print usename is not defined
If we print the username then will throw an error because we again define in if block and it is block-scoped and their scope ends there if we do not again initialize with let in if block then will not throw an error. You can initialize a single time and can modify the value anywhere.
The const works the same as the variable but the main difference is that their value can not be modified within the block or outside the block in this datatype you can add value a single time and will same throughout the execution.
const fullname = "Rakesh Kumar";
console.log(fullname); // Will print Rakesh Kumar
fullname = "Mahesh"; // TypeError: Assignment to constant variable.
Arrow function in JavaScript ES6
It’s the same as a normal function that we create we create as usual like below let’s take an example of the addition of two numbers this function will add two provided numbers and return it.
function add(n1, n2){
return n1+n2;
}
console.log(add(5,2)); // Will print 7
In JavaScript, an arrow function is a shortcut for writing functions. Compared to a conventional function expression, it is syntactically more compact and has several specific features, particularly with regard to this binding. This can be written by using => (“fat arrow”) syntax same function converts into an arrow function. There is also no difference in calling.
const add = (a, b) => {
return a + b;
};
console.log(add(5,2)); // Will print 7
For more convenience, we can also remove “{}” from the function definition. But there is a different calling for single and multiple parameters let see the example for both and single and multiple parameters.
const add = (a, b) => a + b;
console.log(add(5,2)); // Will print 7
const doubler = x => 2 * x;
console.log(doubler(5)); // Will print 10
const say = () => 'Say Hello';
console.log(say()); // Will Say Hello
use case for arrow function:
This implementation in short inline actions, like array operations (map, filter, reduce)
Differences from Regular Functions:
Lexical this Binding: Arrow functions don’t have a context of their own. Rather, they inherit this from the global scope or surrounding function. When you wish to maintain the surrounding scope’s value during callbacks, this is quite helpful.
function counter() {
this.increment = 0;
setInterval(() => {
this.increment++; // 'this' refers to the counter instance
}, 1000);
}
let c = new counter();
console.log(c.age); // will print 0
In the above example, you can see it will print 0 because of this reference to global scope. If we
console.log(this.increment)
within the setInterval curly braces inside then will print. So, it’s globally scoped and block context.
Cannot be used as Constructors:
The arrow function can not be used as a constructor you can see in the above example like
let c = new counter();
But if we can try with same thing with the arrow function will print the below error.
TypeError: counter is not a constructor
Implicit return:
When creating a single argument function then return the keyword not required by default omitted.
const doubler = x => 2 * x;
In this example, you can see I have not used the return keyword.
Use Cases of arrow function in JavaScript ES6
The best implementation of the arrow function you can see in map, filter, and reduce.
Modules in JavaScript ES6
JavaScript modules were established through the ES6 module syntax, providing a standardized approach for importing and exporting code across different files. This modularity enables programmers to organize their code more effectively and facilitates the sharing of functionality among various components of an application.
To explain the module let’s take an example that already stated that modular means we can write code in different files and use any place so let me create three files.user.js // contain user information
address.js //will contain details related to address
main.js // it's the final file in which call both above file code
Exporting from a Module
Default Export:
Now I have created first file user.js
in which you can see it I have saved the user information in variable user and at the end I have added the line export default user;
this code provides the ability to use method, variable, etc in another file. In default export, we can export only the single class, method, and variable in another file by import.
// Filename : user.js
//---------------------------
const user = {
username:"Jhon",
email:"[email protected]"
}
export default user;
Named Exports:
Now move to 2nd file address.js
in which handles user address-related information. Also will see the syntax for named export. To define named export we have to write export preceding to function, variable or class so that can be exported and used in another place. Help of named export we can export multiple function variables and classes.
// Filename : address.js
//---------------------------
export let getCity = () =>{
return "Noida";
}
export let addressId = 25;
Importing from a Module in JavaScript ES6
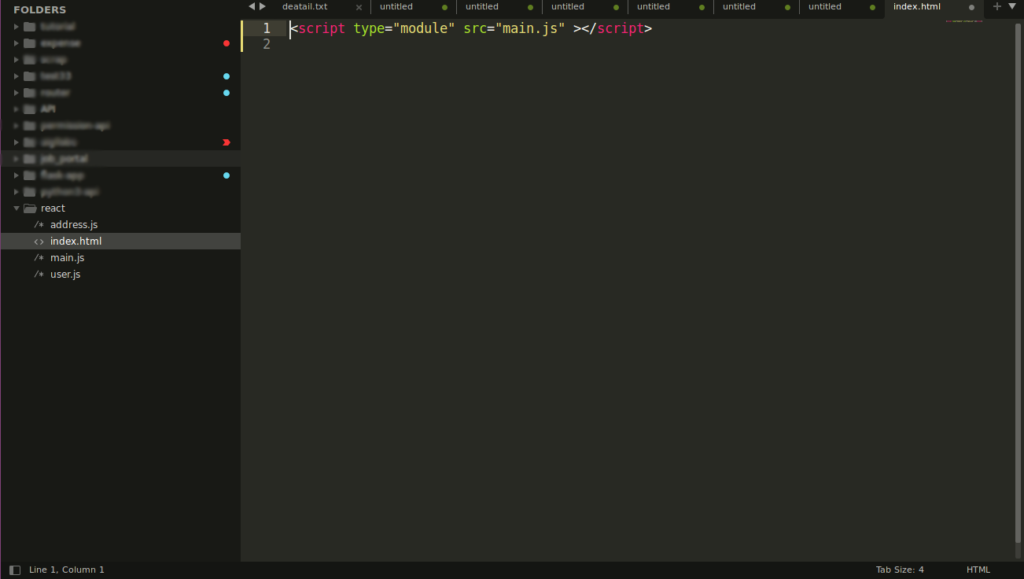
Note:
For importing modules in html file need to add a type module then the module feature will work and the file call should be from a sever like localhost or any server extensions that are available that can be used like Live server which is compatible with Visual Studio Code if you are using Visual Studio Code.
So, now we add the main file main.js
their code explains further. This file will add the main execution and will use the code that is written in the file user.js
and address.js
. So, In the HTML file need to add a statement like below
<script type="module" src="main.js" ></script>
Named Imports:
you can see below code there is added statement import {getCity, addressId} address from './address.js';
In which getCity, addressId
are the function and variable and ./address.js
path of the file in which function and variable are defined.
// Filename : main.js
//---------------------------
import {getCity, addressId} address from './address.js';
console.log(getCity()); // will print Noida
console.log(addressId); // will print 25
Renaming Imports:
For code readability or any purpose if need to rename the name of a function, variable, or class name then in the module feature you can do it same thing explored by below code.
import {addressId as id} from './address.js';
console.log(id) //will print 25
Above code, you can see addressId changed as id and print the same.
Import All:
In the module import, you can import all function methods and variables in a single variable an object by using using * as
so, that no need to import separately same thing explain by code.
import * as address from './address.js';
console.log(address.getCity()); // Will print Noida
console.log(address.addressId); // will print id 25
Default import
Now let me show how can import the default exported module more and less it works like importing all but there is no need to write * as
.
import user from './user.js';
console.log(user.username); // will print Jhon
You can also rename in default import.
import u from './user.js';
console.log(u.username); // will print Jhon
Benefits of Using Modules
- Encapsulation: Modules make code isolated, you can maintain a global namespace more freely by making block-scoped or module-based code.
- Reusability: The same method and variable can be used anywhere there is no need to define and write code again and again.
- Maintainability: Code becomes more organized and readable so that team members or code reviewers can easily understand the code and easy to maintain future.
class & object / oops concept in javascript es6
It is used in approximately all programming languages like PHP, Python, Java, and Javascript. Class-based code has many features like code reusability, readability also helps in memory optimization. It’s a blueprint for creating objects. This feature of javascript es6 is used by many popular frameworks like React, angular, and nextJs. Class code structure definition and calling are the same in all languages only syntax differences are there. Please check the below example for class.
class userDetail{
// Default constructor
constructor(n){
this.name = n;
this.source = "Default";
}
// Individual variable
user_id = 10;
// Arrow function
address = () => {
return {city:"Noida",state:"UP",country:"India"};
}
}
let user = new userDetail("Jhon"); // Set default name by constructor
console.log(user.user_id); // Will print 10
console.log(user.name); // will print Jhon
console.log(user.address().city); // Will print Noida
We have defined a constructor which calls when the class is initialized and we can assign variable value. We can do all things in class that we can do in global space like define arrow function and define normal variables.
Class Inheritance in javascript es6
The code that is already defined or written needs to be reused in the context of inheritance. For example in the class userDetail
the written method and variable need to be used and write the next code then it’s possible by Inheritance. The code extended by keyword extends then adds the class name like extends userDetail
class cart extends userDetail{
getProducts(){
return ["Apple Mobile","Boat Headphone","Mobile Charger"];
}
}
let user = new cart("Jhon"); // Set default name by constructor
console.log(user.user_id); // Will print 10
console.log(user.name); // will print Jhon
console.log(user.getProducts()[0]); // will print Apple Mobile
The variable user_id and name is not defined in the class cart but still print name and user_id because the code of existing class userDetail
added or Inherited in class cart
Array and object Destructuring in in javascript es6
Assigning values from array or object to a variable or you can say unpack values from an array and assign them to variables.
const colors = ['red', 'green', 'blue'];
const [first, second, third] = colors;
console.log(first); // Output: 'red'
console.log(second); // Output: 'green'
console.log(third); // Output: 'blue'
In this, if want skip any variable you can do it by skipping assigned variable like below.
const colors = ['red', 'green', 'blue'];
const [first, second] = colors;
console.log(first); // Output: 'red'
console.log(second); // Output: 'green'
Object Destructuring
You can assign properties to variables by unpacking them from an object utilizing object destructuring. Unless you provide an alternative name, the variable names must correspond to the property names.
const person = { name: 'Alice', age: 25, city: 'New York' };
const { name, age, city } = person;
console.log(name); // Output: 'Alice'
console.log(age); // Output: 25
// You can rename variable in object destructuring
const person = { name: 'Alice', age: 25 };
const { name: personName, age: personAge } = person;
console.log(personName); // Output: 'Alice'
console.log(personAge); // Output: 25
spread and rest operator in javascript es6
Both operators are represented by three dots ...
with the help of these, we can collect the data and spread the next place and also provide flexibility to collect data for function.
Spread Operator
This operator spreads the value of the addition of value to the next variable it works with both array and object. Let’s understand by code.
let carBrand = ["Audi","Ferrai","Hudai"];
let autoBrand = [...carBrand, "Tata", "Nissan"];
console.log(autoBrand); // will print [ 'Audi', 'Ferrai', 'Hudai', 'Tata', 'Nissan' ]
let postAutoBrand = ["Tata", "Nissan", ...carBrand];
console.log(postAutoBrand); // will print [ 'Tata', 'Nissan', 'Audi', 'Ferrai', 'Hudai' ]
In the above code, we have defined three brands in variable carBrand
. Now these three values spread in variable autoBrand by using … and with the use of spread, you can add values at the start and end of indexing.
Rest operator
It also works the same as the rest operator spread operator works for copy values but the rest operator works for the collection of multiple values or data.
Use Case :
Suppose a function needs to pass a dynamic parameter like below.
function userList(user1, user2, user3){
return [user1,user2, user3];
}
console.log(userList("Jhon", "Ram", "Nick")); // [ 'Jhon', 'Ram', 'Nick' ]
console.log(userList("Jhon")); // [ 'Jhon', undefined, undefined ]
You can see that when we did not pass all three parameters in the second call. Then it added undefined on places 2nd and 3rd. So, to fix this issue we have to use the rest operator which collects values in an array format
function userList(...users){
return users;
}
console.log(userList("Jhon", "Ram", "Nick")); // [ 'Jhon', 'Ram', 'Nick' ]
console.log(userList("Jhon")); //[ 'Jhon' ]
Now you can see there is no undefined error the values which you defined will collect it if not then no undefined.
Destructuring with Rest Operator
const fruits = ['apple', 'banana', 'cherry', 'date'];
const [first, second, ...rest] = fruits;
console.log(first); // Output: 'apple'
console.log(second); // Output: 'banana'
console.log(rest); // Output: ['cherry', 'date']
Refrence & premitive type variable assigning
In Primitive type data types (number, string, Symbol, bigint) these are immutable means in this variable the values are directly copied.
let x = 10;
let y = x; // y is now a copy of x's value
y = 20; // changing y does not affect x
console.log(x); // 10
console.log(y); // 20
The reference type data type (array & object) not directly values are copied during variable assign there is referencing the pointer that is in memory it will more clearer from the below example.
let username = { name:"Rakesh Kumar" }
let username2 = username;
console.log(username);// Will print { name: 'Rakesh Kumar' }
console.log(username2); // Will print { name: 'Rakesh Kumar' }
username2.name="Jhon";
console.log(username);// Will print { name: 'Jhon' } but expected Rakesh Kumar
console.log(username2); // Will print { name: 'Jhon' }
In the above example the variable contains the name
“Rakesh Kumar” Before manipulating the variable username2
was print { name: 'Rakesh Kumar' }
but after changing the value in username2.name
both are print { name: 'Jhon' }
but we changed in username2
and username change itself. It happens because both are references to the same location. There is no direct copy of the values.
Now how can we fix this issue so that we can copy the values of one object to another and the original object should be the same or not any modification there? For this, we can use a spread operator.
let username = { name:"Rakesh Kumar" };
let username2 = {...username};
console.log(username);// Will print { name: 'Rakesh Kumar' }
console.log(username2); // Will print { name: 'Rakesh Kumar' }
username2.name="Jhon";
console.log(username);// Will print { name: 'Rakesh Kumar' }
console.log(username2); // Will print { name: 'Jhon' }
Array map and array filter in javascript es6
Array Map:
By giving each element of an existing array a function, the map()
method generates a new array. Every element receives a new value from this method, which map()
then aggregates into the new array. The original array and its values remain unchanged.
const numbers = [1, 2, 3, 4];
const multiplier = numbers.map(num => num * 2);
console.log(multiplier); // [2, 4, 6, 8]
console.log(numbers); // [1, 2, 3, 4] (original values unchanged)
Array filter
The name filter clearly states that the filter()
used for filter the values of the array on the certain business logic or conditions. In this also original array and its values are not changed it creates a new array on the condition true
.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // [2, 4]
console.log(numbers); // [1, 2, 3, 4, 5] (original array remains unchanged)
Conclusion
The complete post is written in keep min in javascript es6. It is a brief discussion about es6 concepts so that you can understand the latest framework and libraries like react, angular, Vue, and react. These front-end technologies are most popular those days but they all use modern javascript concepts. So, I hope you will enjoy the post and try to start learning new frameworks or libraries. Thanks for reading the article.